Project-Based Practice Ideas for Teaching Python Programming in Classroom
Project-based learning can be highly effective for teaching Python programming to high school students, as it engages them in real-world applications and hands-on practice. Here are some examples of projects suitable for grades 9-12, along with the initial code to get started.
1. Simple Calculator
Objective: Create a basic calculator that can perform addition, subtraction, multiplication, and division.
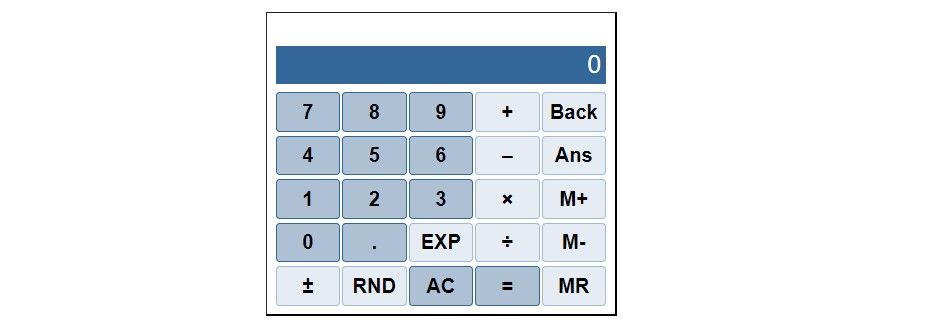
Complete code solution:
# Simple Calculator
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y != 0:
return x / y
else:
return "Error! Division by zero."
print("Select operation:")
print("1.Add")
print("2.Subtract")
print("3.Multiply")
print("4.Divide")
choice = input("Enter choice(1/2/3/4): ")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(num1, "+", num2, "=", add(num1, num2))
elif choice == '2':
print(num1, "-", num2, "=", subtract(num1, num2))
elif choice == '3':
print(num1, "*", num2, "=", multiply(num1, num2))
elif choice == '4':
print(num1, "/", num2, "=", divide(num1, num2))
else:
print("Invalid input")
Output Example:
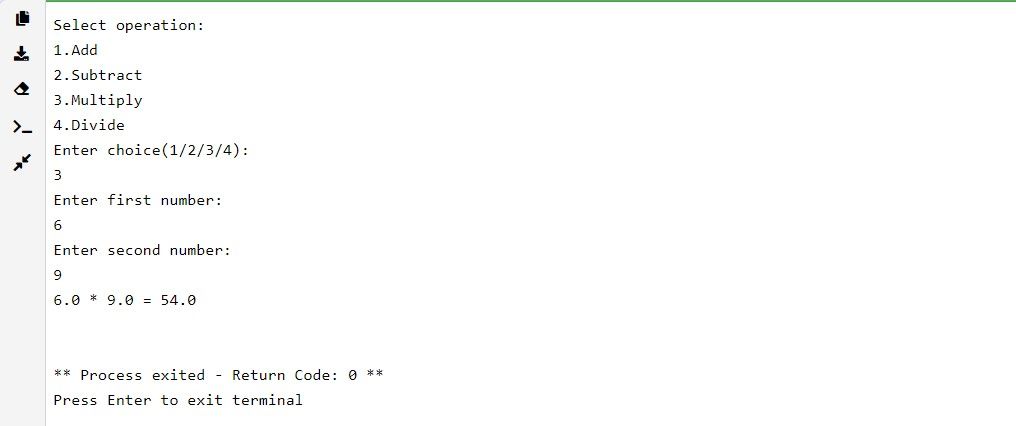
2. Basic Hangman Game
Objective: Develop a hangman game where the player guesses letters to form a word.
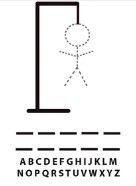
Complete code solution:
import random
def hangman():
words = ['python', 'java', 'kotlin', 'javascript']
word = random.choice(words)
guesses = ''
turns = 10
while turns > 0:
failed = 0
for char in word:
if char in guesses:
print(char, end=' ')
else:
print('_', end=' ')
failed += 1
if failed == 0:
print("\nYou win!")
print("The word is:", word)
break
guess = input("\nGuess a character: ")
guesses += guess
if guess not in word:
turns -= 1
print("Wrong")
print("You have", turns, "more guesses")
if turns == 0:
print("You Lose")
print("The word was:", word)
hangman()
Output Example:
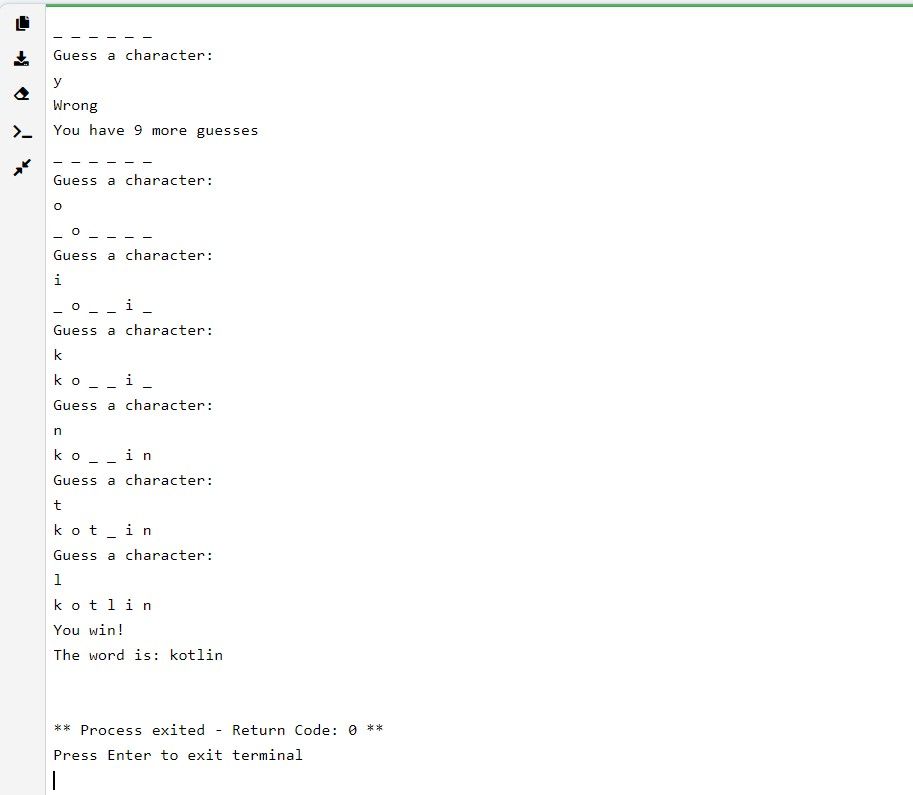
3. Number Guessing Game
Objective: Create a game where the player tries to guess a randomly selected number within a certain range.
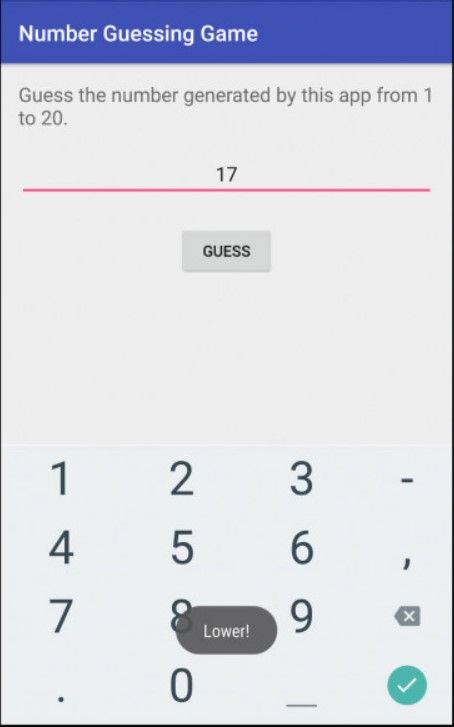
Complete code solution::
import random
def guess_number():
number = random.randint(1, 100)
attempts = 0
while True:
guess = int(input("Guess the number (between 1 and 100): "))
attempts += 1
if guess < number:
print("Too low!")
elif guess > number:
print("Too high!")
else:
print("Congratulations! You guessed the number in", attempts, "attempts.")
break
guess_number()
Output Example:
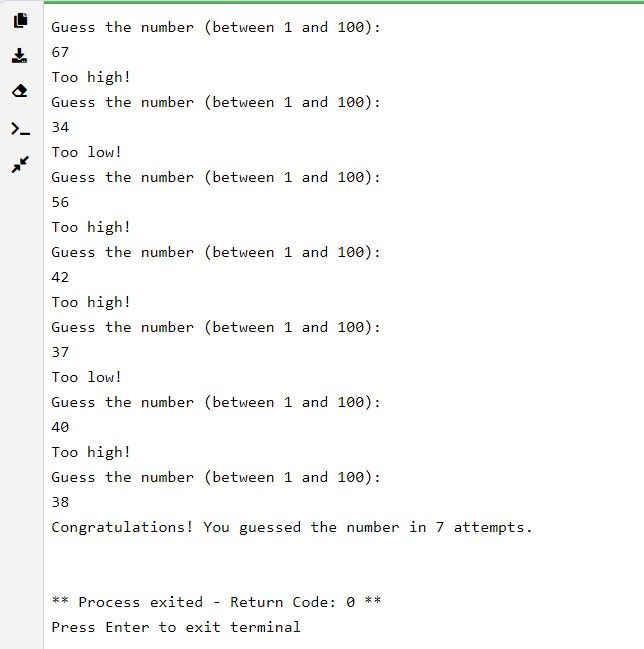
4. To-do List Application
Objective: Build a simple to-do list application to add, remove, and display tasks.
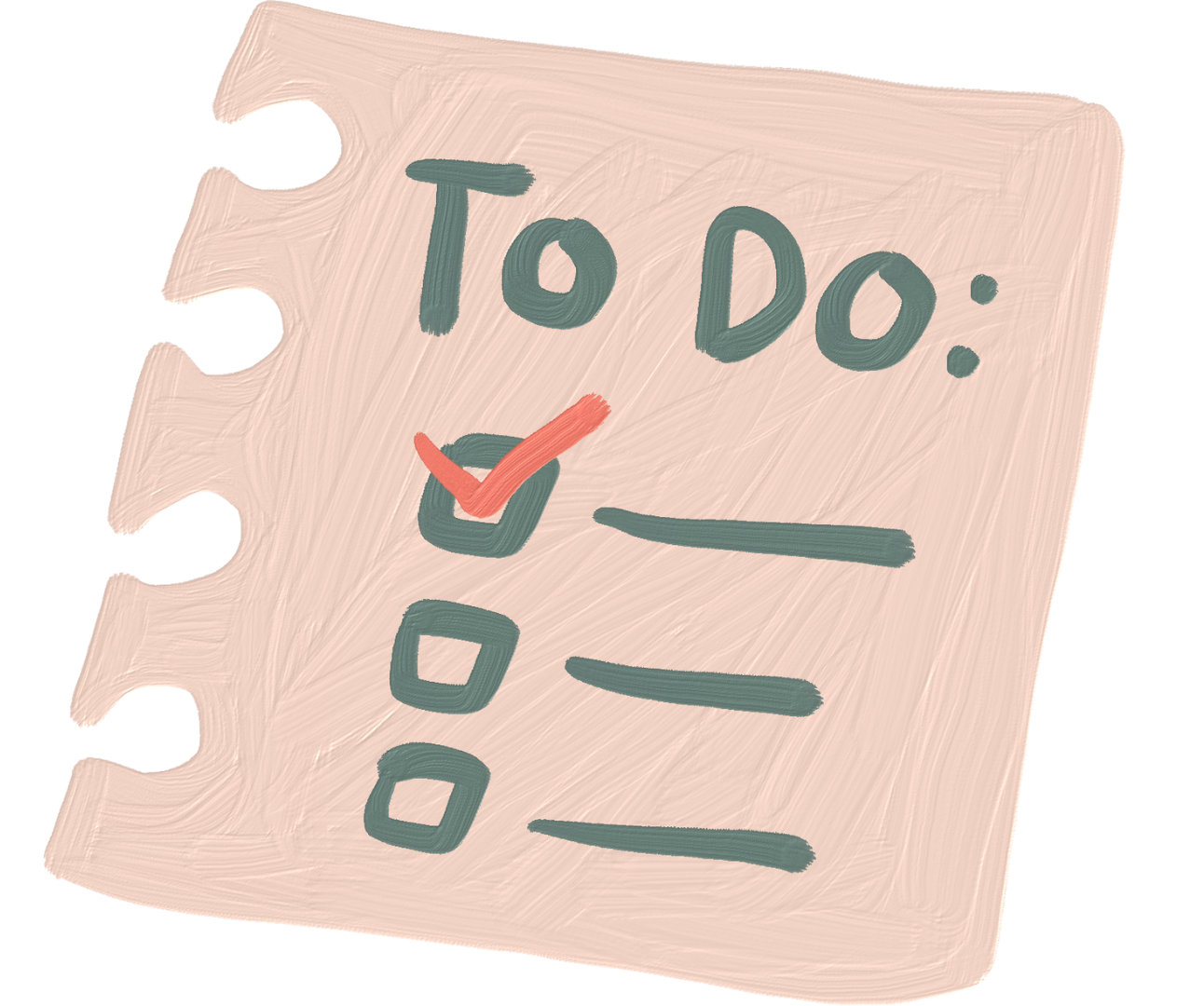
Complete code solution:
tasks = []
def add_task(task):
tasks.append(task)
print(f"Added task: {task}")
def remove_task(task):
if task in tasks:
tasks.remove(task)
print(f"Removed task: {task}")
else:
print(f"Task '{task}' not found")
def display_tasks():
if tasks:
print("Todo List:")
for task in tasks:
print(f"- {task}")
else:
print("No tasks in the todo list")
while True:
print("\n1. Add Task")
print("2. Remove Task")
print("3. Display Tasks")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == '1':
task = input("Enter the task: ")
add_task(task)
elif choice == '2':
task = input("Enter the task to remove: ")
remove_task(task)
elif choice == '3':
display_tasks()
elif choice == '4':
print("Exiting...")
break
else:
print("Invalid choice, please try again.")
Output Example:
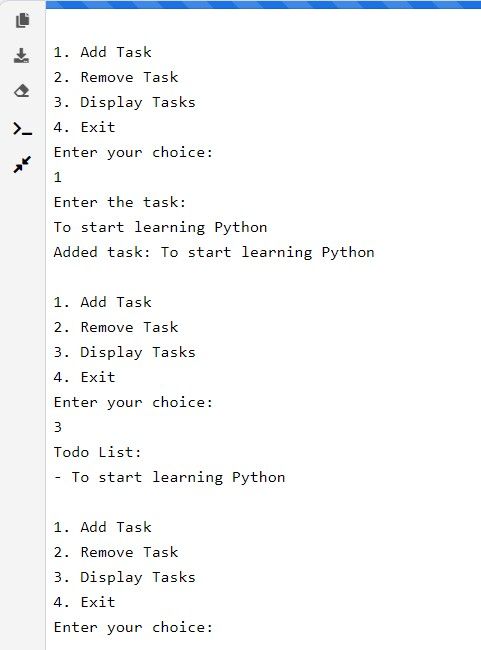
5. Basic Weather App Using an API
Objective: Create a basic weather application that fetches and displays weather data using an API.
Complete code solution:
import requests
def get_weather(city):
api_key = "your_api_key_here" # Replace with your actual API key
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = base_url + "appid=" + api_key + "&q=" + city
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
main = data["main"]
weather = data["weather"][0]
temp = main["temp"]
description = weather["description"]
print(f"Temperature: {temp}")
print(f"Weather Description: {description}")
else:
print("City Not Found")
city = input("Enter city name: ")
get_weather(city)
6. Quiz Game
Objective: Create a quiz game that asks multiple-choice questions and keeps track of the score.
Complete code solution:
def ask_question(question, options, correct_option):
print(question)
for i, option in enumerate(options):
print(f"{i + 1}. {option}")
answer = input("Choose the correct option (1/2/3/4): ")
return int(answer) == correct_option
questions = [
{
"question": "What is the capital of France?",
"options": ["Berlin", "Madrid", "Paris", "Lisbon"],
"correct_option": 3
},
{
"question": "What is 5 + 7?",
"options": ["10", "11", "12", "13"],
"correct_option": 3
}
]
score = 0
for q in questions:
if ask_question(q["question"], q["options"], q["correct_option"]):
print("Correct!")
score += 1
else:
print("Incorrect!")
print(f"Your final score is {score} out of {len(questions)}")
Output example:
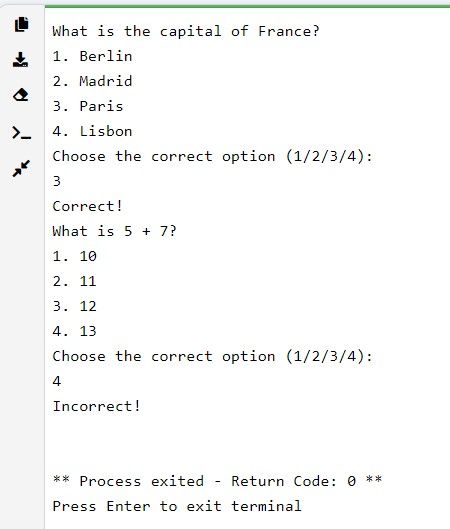
7. Library Management System
Objective: Build a simple library management system that can add, remove, and display books.
Complete code solution:
library = []
def add_book(title, author):
book = {"title": title, "author": author}
library.append(book)
print(f"Added book: {title} by {author}")
def remove_book(title):
global library
library = [book for book in library if book["title"] != title]
print(f"Removed book: {title}")
def display_books():
if library:
print("Library Books:")
for book in library:
print(f"Title: {book['title']}, Author: {book['author']}")
else:
print("No books in the library")
while True:
print("\n1. Add Book")
print("2. Remove Book")
print("3. Display Books")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == '1':
title = input("Enter book title: ")
author = input("Enter book author: ")
add_book(title, author)
elif choice == '2':
title = input("Enter the book title to remove: ")
remove_book(title)
elif choice == '3':
display_books()
elif choice == '4':
print("Exiting...")
break
else:
print("Invalid choice, please try again.")
Output example:
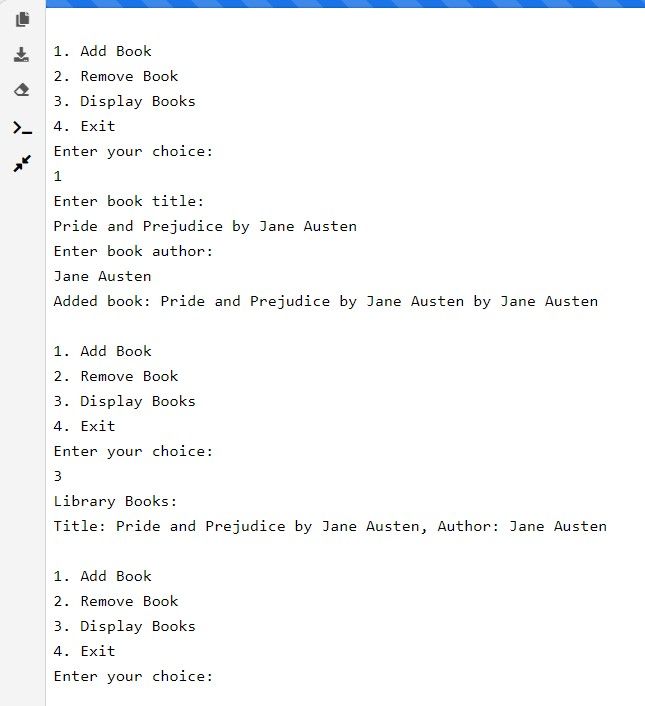
8. Personal Expense Tracker
Objective: Create an expense tracker that logs expenses and displays the total expenditure.
Complete code solution:
expenses = []
def add_expense(description, amount):
expense = {"description": description, "amount": amount}
expenses.append(expense)
print(f"Added expense: {description} - ${amount}")
def display_expenses():
total = 0
print("Expenses:")
for expense in expenses:
print(f"{expense['description']}: ${expense['amount']}")
total += expense['amount']
print(f"Total expenditure: ${total}")
while True:
print("\n1. Add Expense")
print("2. Display Expenses")
print("3. Exit")
choice = input("Enter your choice: ")
if choice == '1':
description = input("Enter expense description: ")
amount = float(input("Enter expense amount: "))
add_expense(description, amount)
elif choice == '2':
display_expenses()
elif choice == '3':
print("Exiting...")
break
else:
print("Invalid choice, please try again.")
Output example:
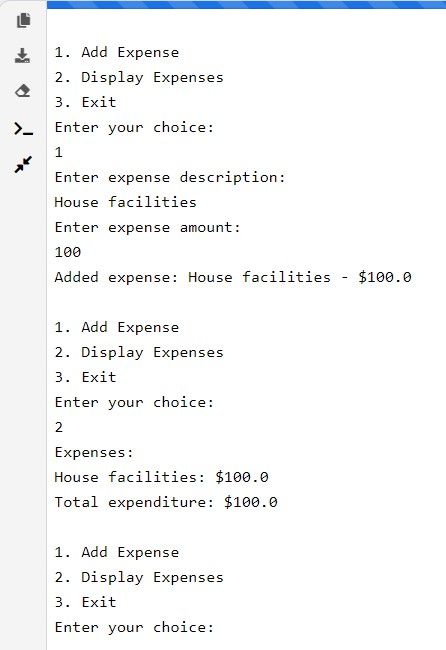
9. Tic-Tac-Toe Game
Objective: Develop a two-player tic-tac-toe game.
Complete code solution:
board = [' ' for _ in range(9)]
def print_board():
for i in range(3):
print(f"{board[3*i]} | {board[3*i+1]} | {board[3*i+2]}")
if i < 2:
print("---------")
def check_winner(player):
win_conditions = [(0,1,2), (3,4,5), (6,7,8), (0,3,6), (1,4,7), (2,5,8), (0,4,8), (2,4,6)]
for condition in win_conditions:
if board[condition[0]] == board[condition[1]] == board[condition[2]] == player:
return True
return False
def play_game():
current_player = 'X'
for _ in range(9):
print_board()
move = int(input(f"Player {current_player}, enter your move (1-9): ")) - 1
if board[move] == ' ':
board[move] = current_player
if check_winner(current_player):
print_board()
print(f"Player {current_player} wins!")
return
current_player = 'O' if current_player == 'X' else 'X'
else:
print("Invalid move, try again.")
print_board()
print("It's a tie!")
play_game()
Output example:
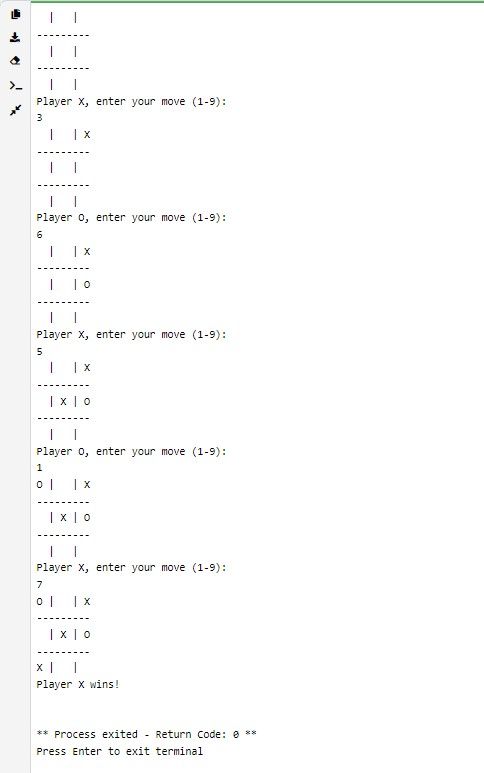
10. Weather Notifier with Email
Objective: Create a program that checks the weather and sends an email notification if certain conditions are met.
Complete code solution:
import requests
import smtplib
def get_weather(city):
api_key = "your_api_key_here" # Replace with your actual API key
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = base_url + "appid=" + api_key + "&q=" + city
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
main = data["main"]
weather = data["weather"][0]
return main["temp"], weather["description"]
else:
return None, None
def send_email(subject, body):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login("your_email@gmail.com", "your_password") # Replace with your actual email and password
message = f"Subject: {subject}\n\n{body}"
server.sendmail("your_email@gmail.com", "recipient_email@gmail.com", message) # Replace with recipient email
server.quit()
city = "London"
temp, description = get_weather(city)
if temp and description:
if "rain" in description.lower():
subject = "Weather Alert"
body = f"It's currently raining in {city}. Temperature: {temp}K."
send_email(subject, body)
11. Simple Contact Book
A project to introduce dictionaries, file handling, and basic data management.
Complete code solution:
contacts = {}
def display_contacts():
print("\nContacts:")
for name, number in contacts.items():
print(f"{name}: {number}")
def add_contact(name, number):
contacts[name] = number
print(f"Contact '{name}' added.")
def remove_contact(name):
if name in contacts:
del contacts[name]
print(f"Contact '{name}' removed.")
else:
print(f"No contact found with the name '{name}'.")
while True:
print("\nOptions:")
print("1. Display Contacts")
print("2. Add Contact")
print("3. Remove Contact")
print("4. Quit")
choice = input("Enter choice (1/2/3/4): ")
if choice == '1':
display_contacts()
elif choice == '2':
name = input("Enter contact name: ")
number = input("Enter contact number: ")
add_contact(name, number)
elif choice == '3':
name = input("Enter contact name to remove: ")
remove_contact(name)
elif choice == '4':
break
else:
print("Invalid choice, please try again.")
Output example:
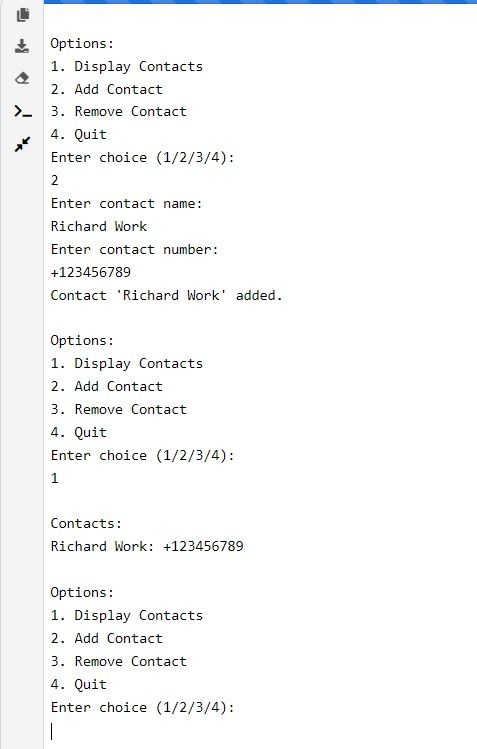
12. Chatbot
Objective: Develop a simple chatbot that can respond to user inputs.
Complete code solution:
def chatbot():
print("Hello! I am a chatbot. How can I help you?")
while True:
user_input = input("You: ")
if user_input.lower() in ['hi', 'hello']:
print("Chatbot: Hello! How are you?")
elif user_input.lower() in ['bye', 'exit']:
print("Chatbot: Goodbye!")
break
else:
print("Chatbot: I don't understand that.")
chatbot()
Output example:
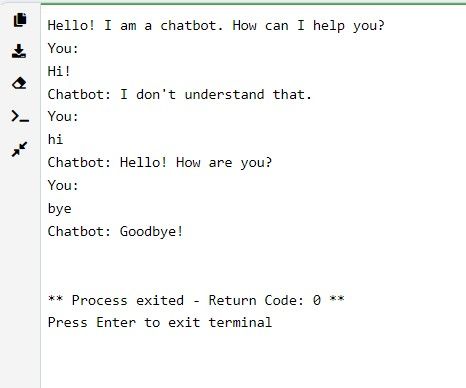
13. Currency Converter
Objective: Create a currency converter that converts between different currencies using an API.
Complete code solution:
import requests
def get_exchange_rate(base_currency, target_currency):
url = f"https://api.exchangerate-api.com/v4/latest/{base_currency}"
response = requests.get(url)
data = response.json()
return data['rates'][target_currency]
def convert_currency(amount, base_currency, target_currency):
rate = get_exchange_rate(base_currency, target_currency)
return amount * rate
base_currency = input("Enter base currency: ").upper()
target_currency = input("Enter target currency: ").upper()
amount = float(input(f"Enter amount in {base_currency}: "))
converted_amount = convert_currency(amount, base_currency, target_currency)
print(f"{amount} {base_currency} = {converted_amount:.2f} {target_currency}")
14. Password Generator
Objective: Develop a program that generates random passwords based on user-specified criteria.
Complete code solution:
import random
import string
def generate_password(length, include_uppercase, include_numbers, include_special):
characters = string.ascii_lowercase
if include_uppercase:
characters += string.ascii_uppercase
if include_numbers:
characters += string.digits
if include_special:
characters += string.punctuation
password = ''.join(random.choice(characters) for _ in range(length))
return password
length = int(input("Enter password length: "))
include_uppercase = input("Include uppercase letters? (y/n): ").lower() == 'y'
include_numbers = input("Include numbers? (y/n): ").lower() == 'y'
include_special = input("Include special characters? (y/n): ").lower() == 'y'
password = generate_password(length, include_uppercase, include_numbers, include_special)
print(f"Generated password: {password}")
Output example:
15. Basic Web Scraper
Objective: Create a web scraper to extract and display information from a webpage.
Complete code solution:
import requests
from bs4 import BeautifulSoup
def scrape_website(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for heading in soup.find_all('h2'):
print(heading.text.strip())
url = "https://www.example.com" # Replace with a real URL
scrape_website(url)
Output example:
16. Rock, Paper, Scissors Game
Objective: Create a rock, paper, scissors game that can be played against the computer.
Complete code solution:
import random
def get_computer_choice():
choices = ['rock', 'paper', 'scissors']
return random.choice(choices)
def get_winner(player, computer):
if player == computer:
return "It's a tie!"
elif (player == 'rock' and computer == 'scissors') or (player == 'paper' and computer == 'rock') or (player == 'scissors' and computer == 'paper'):
return "You win!"
else:
return "You lose!"
while True:
player_choice = input("Enter rock, paper, or scissors (or 'exit' to quit): ").lower()
if player_choice == 'exit':
break
if player_choice not in ['rock', 'paper', 'scissors']:
print("Invalid choice, please try again.")
continue
computer_choice = get_computer_choice()
print(f"Computer chose: {computer_choice}")
print(get_winner(player_choice, computer_choice))
Output example:
17. Simple Voting System
Objective: Develop a simple voting system that records and displays votes for different options.
Complete code solution:
votes = {"Option A": 0, "Option B": 0, "Option C": 0}
def vote(option):
if option in votes:
votes[option] += 1
else:
print("Invalid option!")
def display_results():
for option, count in votes.items():
print(f"{option}: {count} votes")
while True:
print("\nOptions: A, B, C")
choice = input("Enter your vote (or 'exit' to quit): ").upper()
if choice == 'EXIT':
break
vote("Option " + choice)
display_results()
Output example:
18. Stopwatch
Objective: Create a simple stopwatch using the time module.
Complete code solution:
import time
def stopwatch():
print("Press ENTER to start the stopwatch and press ENTER again to stop it.")
input()
start_time = time.time()
input()
end_time = time.time()
elapsed_time = end_time - start_time
print(f"Elapsed time: {elapsed_time:.2f} seconds")
stopwatch()
Output example:
Where can students write these projects?
These code editors and IDEs, listed in our article, provide various features to cater to different needs, making them suitable for both educational purposes and professional development.