A Brief Overview of Essential Python Libraries and Frameworks to Use for Beginners
Python's popularity is largely due to its rich ecosystem of libraries and frameworks that simplify and enhance programming tasks. For beginners, familiarizing yourself with some of these key libraries and frameworks can significantly boost your productivity and help you tackle a wide range of projects. Here’s a guide to some of the most important Python libraries and frameworks for beginners.
Libraries
1. NumPy
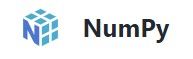
NumPy (Numerical Python) is a fundamental library for numerical computations. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Key Features:
- ndarray: Efficient array manipulation, providing a powerful N-dimensional array object.
- Broadcasting: Handles operations between arrays of different shapes.
- Linear Algebra: Comprehensive functions for linear algebra, random number generation, and more.
Use Cases:
- Scientific Computing: Essential for handling numerical data in scientific research.
- Data Analysis: Basis for data manipulation and analysis, often used alongside Pandas.
- Machine Learning: Core data structure for machine learning algorithms.
- Why It's Useful: Essential for scientific computing and data manipulation.
Getting Started:
import numpy as np
# Create a 2x3 array
array = np.array([[1, 2, 3], [4, 5, 6]])
print(array)
Resources:
2. Pandas
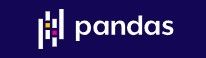
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames, which are akin to tables in a database or Excel sheet, making it easier to handle and analyze structured data.
Key Features:
- DataFrames: Tabular data structures similar to Excel sheets or SQL tables.
- Data Cleaning: Tools for handling missing data, filtering, and transforming datasets.
- Integration: Easily integrates with other libraries like NumPy and Matplotlib for data analysis and visualization.
Use Cases:
- Data Cleaning: Preprocessing data for analysis or machine learning.
- Exploratory Data Analysis: Quickly summarizing data to discover patterns and insights.
- Time Series Analysis: Handling time-indexed data for various analyses.
Getting Started:
import pandas as pd
# Create a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Resources:
3. Matplotlib
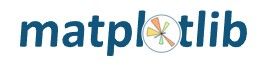
Matplotlib is a plotting library that enables the creation of static, animated, and interactive visualizations in Python. It is particularly useful for generating graphs and charts from data.
Key Features:
- Plotting Functions: Tools for creating various types of plots (line, bar, scatter, histogram, etc.).
- Customization: Extensive options to customize the appearance of plots.
- Integration: Works seamlessly with other data handling libraries.
Use Cases:
- Data Visualization: Creating visual representations of data to uncover trends and patterns.
- Scientific Visualization: Plotting scientific data for analysis and presentation.
- Interactive Plots: Generating interactive plots for web applications or exploratory analysis.
Getting Started:
import matplotlib.pyplot as plt
# Create a simple plot
plt.plot([1, 2, 3, 4], [10, 20, 25, 30])
plt.title('Simple Plot')
plt.xlabel('x')
plt.ylabel('y')
plt.show()
Resources:
4. Requests
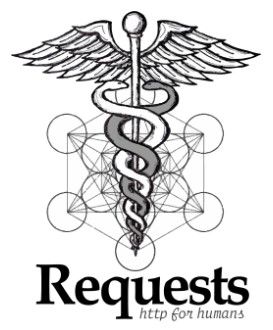
Requests is a simple and elegant library for making HTTP requests. It abstracts the complexities of making requests behind a simple API, allowing you to send HTTP requests with ease.
Key Features:
- Simplified HTTP Requests: Send all types of HTTP requests (GET, POST, PUT, DELETE) with simple syntax.
- Response Handling: Easy handling of response data, including JSON, text, and binary.
- Session Objects: Manage and persist settings across multiple requests.
Use Cases:
- API Interaction: Accessing and interacting with web APIs.
- Web Scraping: Fetching data from websites for analysis.
- Automation: Automating web interactions and data retrieval.
Getting Started:
import requests
# Make a GET request
response = requests.get('https://api.github.com')
print(response.status_code)
Resources:
Frameworks
1. Django
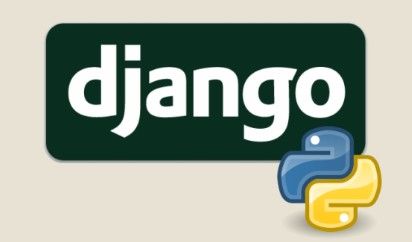
Django is a high-level web framework that encourages rapid development and clean, pragmatic design. It follows the "batteries-included" philosophy, providing many features out of the box.
Key Features:
- ORM (Object-Relational Mapping): Simplifies database interactions by mapping database tables to Python objects.
- Admin Interface: Automatically generated admin interface for managing application data.
- Security: Built-in security features to protect against common web threats.
Use Cases:
- Web Development: Building robust, scalable web applications.
- API Development: Creating RESTful APIs with Django REST Framework.
- E-commerce: Developing e-commerce platforms and online stores.
Getting Started:
# Install Django
pip install django
# Create a new Django project
django-admin startproject myproject
cd myproject
# Run the development server
python manage.py runserver
Resources:
2. Flask
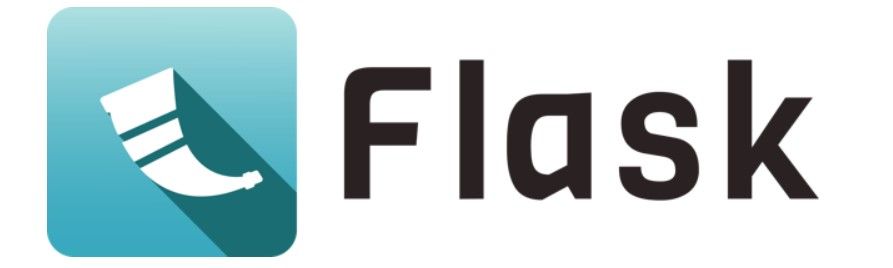
Flask is a lightweight web framework that provides the essentials for web development without imposing a specific structure or dependencies. It’s known for its simplicity and flexibility.
Key Features:
- Minimalism: Lightweight and easy to get started with, making it ideal for small to medium-sized applications.
- Extensibility: Easily extendable with various plugins and extensions.
- Simplicity: Simple and intuitive API for rapid development.
Use Cases:
- Web Development: Building simple, yet powerful web applications.
- Prototyping: Quickly developing prototypes and small-scale projects.
- API Development: Creating RESTful APIs.
Getting Started:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
Resources:
3. Scrapy
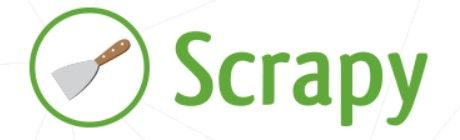
Scrapy is a powerful and fast high-level web crawling and web scraping framework. It is used to extract data from websites and process them as needed.
Key Features:
- Efficiency: Built for speed and efficiency in scraping large websites.
- Extensibility: Highly customizable with middlewares and pipelines.
- Built-in Tools: Tools for handling various tasks like parsing, scheduling, and exporting data.
Use Cases:
- Web Scraping: Extracting data from websites for analysis.
- Data Mining: Collecting large datasets from the web.
- Web Crawling: Automated browsing and data collection from websites.
Getting Started:
# Install Scrapy
pip install scrapy
# Create a new Scrapy project
scrapy startproject myproject
cd myproject
# Generate a spider
scrapy genspider example example.com
Resources:
4. Keras
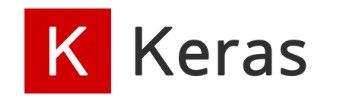
Keras is a high-level neural networks API, written in Python and capable of running on top of TensorFlow, CNTK, or Theano. It is user-friendly, modular, and extensible, making it ideal for beginners in deep learning.
Key Features:
- User-Friendly: Simple and consistent interface optimized for common use cases.
- Modular: A model-building process that is highly modular, allowing for the easy addition of layers and loss functions.
- Scalability: Runs seamlessly on CPUs and GPUs.
Use Cases:
- Machine Learning: Building and training deep learning models.
- Artificial Intelligence: Developing AI applications, from simple neural networks to complex models.
- Research: Prototyping new deep learning algorithms and architectures.
Getting Started:
from keras.models import Sequential
from keras.layers import Dense
# Define a simple neural network
model = Sequential()
model.add(Dense(32, input_dim=784, activation='relu'))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(loss='categorical_crossentropy', optimizer='sgd', metrics=['accuracy'])
Resources:
Conclusion
These libraries and frameworks provide a solid foundation for beginners to start their Python programming journey. Whether you’re interested in data analysis, web development, or machine learning, these tools will help you get started and build your skills.
Happy coding!